快速入门Sass:Sass中的体系结构
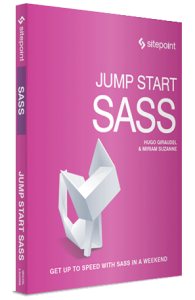
The following is a short extract from our recent book, Jump Start Sass, available for free to SitePoint Premium members. Print copies are sold in stores worldwide, or you can order them here. We hope you enjoy this extract and find it useful.
以下是我们最近的书Jump Start Sass的简短摘录, SitePoint Premium会员可以免费获得。 印刷版在世界各地的商店都有出售,您也可以在此处订购 。 我们希望您喜欢此摘录并觉得有用。
Architecture has always been one of the major pain points in CSS development. Without any variables, control directives, macros, or object inheritance, CSS code tends to be long and repetitive-a single ever-growing file. While it’s technically possible to split plain CSS into multiple files that reference each other with @import
, the additional HTTP requests make that a poor solution. As you’ve seen, Sass has an answer for every piece of the architecture puzzle-but what’s the best way to put it all together?
架构一直是CSS开发的主要痛点之一。 没有任何变量,控制指令,宏或对象继承,CSS代码往往又长又重复-一个不断增长的文件。 从技术上讲,可以将纯CSS拆分为多个文件,这些文件可以使用@import
相互引用,但其他HTTP请求使该解决方案不可行。 如您所见,Sass可以解决所有架构难题,但是将其整合在一起的最佳方法是什么?
Ask ten experts, and you’ll receive ten different answers-most of them involving (or aided by) Sass. OOCSS, SMACSS, Atomic Design, ITCSS, and BEM are all popular systems for CSS architecture, but there are many more. If you’re using a front-end framework such as Bootstrap or Foundation, there might be some architectural opinions already built in.
询问十位专家,您将收到十个不同的答案-其中大多数涉及Sass(或由Sass辅助)。 OOCSS , SMACSS , Atomic Design , ITCSS和BEM都是CSS架构的流行系统,但还有更多。 如果您使用的是诸如Bootstrap或Foundation之类的前端框架,则可能已经内置了一些体系结构意见。
These are all solid systems, none of which were designed with your project in mind. CSS architecture is hard, so it’s a mistake to trust any one-size-fits-all solution. There is no “right” answer that works for every team on every project. We’d recommend learning them all, and then mashing together the best parts to create a system that works well for you.
这些都是可靠的系统,没有一个是为您的项目而设计的。 CSS体系结构很难,因此信任任何一种千篇一律的解决方案都是一个错误。 没有“正确”的答案适用于每个项目的每个团队。 我们建议您学习所有这些内容,然后将最好的部分融合在一起,以创建一个适合您的系统。
Let’s start with a broad discussion of the building blocks, and then look at the ways we can fit them together.
让我们从对构建基块的广泛讨论开始,然后看看我们如何将它们组合在一起。
多个文件和文件夹 ( Multiple Files and Folders)
Breaking your code into multiple files is one key advantage to using a preprocessor, and forms the basis of any architecture. With Sass, there’s no harm in breaking your code into the smallest logical units and organizing it into multiple files and folders. We recommend taking full advantage of it.
将代码分为多个文件是使用预处理器的主要优势之一,并且构成任何体系结构的基础。 使用Sass,将代码分解为最小的逻辑单元并将其组织为多个文件和文件夹没有任何害处。 我们建议充分利用它。
Sass has bestowed new power on the CSS @import
rule, allowing you to combine Sass and CSS files during compilation so they can be sent to the browser as one single file. This is the only place where Sass has stepped on the toes of an existing CSS directive, so it behaves differently in Sass than it did in CSS.
Sass在CSS @import
规则上赋予了新的功能,使您可以在编译期间合并Sass和CSS文件,以便它们可以作为一个文件发送到浏览器。 这是Sass踩到现有CSS指令的唯一位置,因此它在Sass中的行为与在CSS中的行为有所不同。
CSS导入 ( CSS Imports)
As mentioned, the CSS @import
directive allows you to reference one CSS file from another. Importing is handled by the browser and requires additional HTTP requests-since the importing file has to be parsed before the @import
directive is discovered. If you have a chain of files importing each other, those imports will happen in sequence, blocking the document from rendering until all the CSS has loaded. For that reason, most people avoid CSS imports entirely.
如前所述,CSS @import
指令允许您从另一个引用一个CSS文件。 导入由浏览器处理,并且需要其他HTTP请求-因为必须在发现@import
指令之前解析导入文件。 如果您有一连串的文件导入,这些导入将按顺序进行,从而阻止文档呈现,直到所有CSS都已加载。 因此,大多数人完全避免CSS导入。
Using CSS imports, you can reference another CSS file using relative or absolute paths, even adding a media query rule for conditional imports. Even though Sass provides different functionality under the same at-rule, there are various cases in which Sass will fall back to the vanilla CSS output, such as when:
使用CSS导入,您可以使用相对或绝对路径引用另一个CSS文件,甚至可以为条件导入添加媒体查询规则。 尽管Sass在相同规则下提供了不同的功能,但在各种情况下Sass都会退回到原始CSS输出,例如:
an imported file has a
.css
extension导入的文件的扩展名为
.css
a filename begins with
http://
orhttps://
文件名以
http://
或https://
开头the filename is a
url(..)
function文件名是
url(..)
函数@import
has any media queries@import
有任何媒体查询
The following will compile to standard CSS imports, even in Sass:
以下内容将编译为标准CSS导入,即使在Sass中也是如此:
@import 'relative/styles.css';
@import 'http://absolute.com/styles.css';
@import url('landscape.css') screen and (orientation: landscape);
Sass导入和部分 ( Sass Imports and Partials)
Sass imports look similar to CSS imports, but the imported files are compiled into one single output file, as though their contents (including variables, mixins, functions, and placeholders) were copied and pasted into place before compilation. This type of Sass import will only work on files with .sass or .scss extensions, but you can leave the extension off when importing (as long as there are no similarly named files). In fact, we recommend dropping the extension whenever you can, for simplicity. It’s also possible to import multiple files in one command, or import files into a nested context:
Sass导入看起来类似于CSS导入,但是导入的文件被编译到一个输出文件中,就好像它们的内容(包括变量,mixin,函数和占位符)在编译之前已被复制并粘贴到位。 这种类型的萨斯进口只会与.sass或.scss扩展名的文件的工作,但你可以离开扩展导入时关闭(只要不存在类似命名的文件)。 实际上,为简单起见,我们建议您尽可能删除该扩展名。 也可以在一个命令中导入多个文件,或将文件导入嵌套的上下文中:
// Import an explicit file relative to the current directory
@import 'path/to/explicit.scss';
// Import a file with either the .sass or .scss extension
@import 'implicit';
// Import multiple files...
@import 'path/to/emory.scss', 'miko', 'path/to/gracie';
// Import a file into a nested context...
// (imagine the file copied and pasted into this context)
.latte {
@import 'espresso';
}
The most common use of Sass importing is for partial files—Sass files that are not compiled on their own but are for importing into other files. If you want a Sass file to remain uncompiled until it’s imported, add an underscore (_) to the start of the filename. Sass files that start with _ won’t compile on their own, but can be imported into other files. When importing partials, Sass allows you to leave the _ off, which is similar to leaving off an extension. For example:
Sass导入的最常见用途是用于部分文件-Sass文件不是自己编译的,而是用于导入其他文件的。 如果您希望Sass文件在导入之前保持未编译状态,请在文件名的开头添加下划线( _ )。 以_开头的Sass文件不会自行编译,但可以导入到其他文件中。 导入部分时,Sass允许您关闭_ ,这类似于省略扩展名。 例如:
// _authors.scss
.miriam { background: blue; }
// jumpstartsass.scss
@import 'authors'; // Shorthand for importing '_authors.scss'
// jumpstartsass.css (compiled CSS)
.miriam { background: blue; }
Running Sass in this directory (sass --update .
) compiles jumpstartsass.scss to jumpstartsass.css; however, it won’t create an _authors.css file, since it has a leading underscore.
在此目录( sass --update .
)中运行Sass sass --update .
jumpstartsass.scss编译为jumpstartsass.css ; 但是,它不会创建_authors.css文件,因为它具有下划线。
Sass partials form the basis of any Sass architecture. Because all Sass imports are handled at compile time and never interrupt the browser, it’s perfectly safe (and recommended) to use as many partials as necessary, compiling them into a single stylesheet for production. For the sake of being organized we recommend breaking out partials liberally, sorting them into folders, and importing them all back into one single master file for compilation. A common Sass directory for a project might look like this:
Sass局部构成任何Sass体系结构的基础。 由于所有Sass导入都是在编译时处理的,并且永远不会中断浏览器,因此,根据需要使用尽可能多的部分并将它们编译为一个样式表以进行生产是绝对安全的(并建议)。 为了有条理,我们建议自由地分解部分,将它们分类到文件夹中,然后将所有部分重新导入到一个主文件中进行编译。 项目的公共Sass目录可能如下所示:
sass/
|
|– config/
| |– _colors.scss # Color palettes
| |– _webfonts.scss # Webfont information
| … # Etc.
|
|– layout/
| |– _navigation.scss # Navigation
| |– _banner.scss # Site Banner
| … # Etc.
|
|– modules/
| |– _calendar.scss # Calendar widget styles
| |– _contact.scss # Contact form styles
| … # Etc.
|
|– patterns/
| |– _buttons.scss # Buttons
| |– _dropdown.scss # Dropdown
| … # Etc.
|
|- main.scss # The primary Sass file to be compiled
After organizing all your partials, they can be imported into the single primary main.scss file for compilation:
组织完所有部分之后,可以将它们导入到单个主main.scss文件中进行编译:
// Primary Sass File: main.scss
@import 'config/colors';
@import 'config/webfonts';
@import 'patterns/buttons';
@import 'patterns/dropdown';
@import 'layout/navigation';
@import 'layout/banner';
@import 'modules/calendar';
@import 'modules/contact';
组成和组织 (Components and Organization)
We’ve advised you to use partials, folders, and imports—but what’s really important is how to use them efficiently. This is where everyone’s opinions differ, and your mileage may vary.
我们建议您使用部分,文件夹和导入-但是真正重要的是如何有效地使用它们。 这是每个人的意见都不同的地方,您的里程可能会有所不同。
Most CSS and Sass organization systems are based on some concept of user interface “components” or discrete pieces that can be put together to form a complete project. Components can be any size or shape, but they should focus on doing one task independently, and in a reusable way. A button, a drop-down, a calendar, and a search form are all examples of components that can be reused at different places across a project. Thinking about your project as a collection of components will help you towards having an organized and maintainable architecture, whether you’re using Sass or plain CSS.
大多数CSS和Sass组织系统都是基于用户界面“组件”或离散组件的某些概念,这些概念可以组合在一起形成一个完整的项目。 组件可以是任何大小或形状,但它们应专注于以可重用的方式独立完成一项任务。 按钮,下拉菜单,日历和搜索表单都是可以在项目中不同位置重用的组件示例。 无论您使用的是Sass还是普通CSS,将您的项目视为组件的集合都将帮助您建立一个有组织且可维护的体系结构。
Because of the way CSS works, the order of your code will also affect its meaning: later code has priority in the cascade over the code before it. Some of the popular branded architectures (the ones you know by name) try to eliminate this feature of the cascade entirely, but I use it as a guide—organizing code from the most general to the most specific—so the priority override makes sense. Code that we want applied generally across the site should come first, growing slowly in specificity and detail as we move towards more unique components and special cases.
由于CSS的工作方式,代码的顺序也会影响其含义:后面的代码在级联中优先于之前的代码。 一些流行的品牌体系结构(您已经知道的名称)试图完全消除级联的这一功能,但是我将其用作指导-从最一般到最具体的组织代码-因此优先级覆盖才有意义。 首先,我们要在整个站点上普遍应用的代码应排在第一位,随着我们朝着更加独特的组件和特殊情况迈进,其特异性和详细性将缓慢增长。
I first learned of this approach from Natalie Downe’s wonderful CSS Systems talk in 2008 before I’d ever used Sass. Her architecture at the time started with elements (h2
, ol
, ul
, and so on) grouped by “type”, followed by classes grouped by the “effect” created, and finally IDs grouped by the “component” they affect. These days it’s common practice to avoid IDs altogether, and break elements into smaller pieces, but the concept remains the same: global defaults first, followed by site-wide patterns and broad layouts, and finally, more specific modules, themes, and overrides.
在我使用Sass之前,我从2008年Natalie Downe精彩的CSS Systems演讲中学到了这种方法。 当时,她的体系结构从按“类型”分组的元素( h2
, ol
, ul
等)开始,然后是按创建的“效果”分组的类,最后是按它们影响的“组件”分组的ID。 如今,通常的做法是完全避免使用ID,并将元素分成较小的部分,但概念仍然保持不变:首先是全局默认值,然后是整个站点范围的模式和广泛的布局,最后是更具体的模块,主题和替代。
Sass projects include another category of site-wide defaults not found in CSS: code with no output at all—such as variables, functions, and mixin definitions. Many people (myself included) break that code into its own set of partials, to be imported anywhere it might be useful. I have a complete folder just for site-wide Sass helpers and configuration that don’t result in output. Those files act as a single, definitive, and reusable configuration that defines the boundaries of a project. By ensuring your configuration is output-free, you can import it anywhere without worrying about duplicated or unwanted styles.
Sass项目包括CSS中找不到的另一类站点范围默认值:根本没有输出的代码,例如变量,函数和混合定义。 许多人(包括我自己在内)将代码分解成自己的部分代码,然后将其导入可能有用的任何位置。 我有一个仅用于站点范围内的Sass助手和配置的完整文件夹,不会导致输出。 这些文件充当定义项目边界的单一,确定且可重用的配置。 通过确保您的配置没有输出,您可以将其导入任何地方,而不必担心样式重复或多余。
Here are some guidelines for thinking about architecture:
以下是有关架构的一些指导原则:
Break your code into the smallest logical component partials.
将您的代码分成最小的逻辑组件部分。
Organize your partials into grouped folders based on specificity.
根据具体情况将局部文件组织到分组文件夹中。
Import those partials into one master file in order of specificity.
按特定顺序将这些部分导入一个主文件中。
However, many variations do exist on the specific ways people implement those ideas.
但是,人们在实现这些想法的具体方式上确实存在许多变化。
You may also find that a lot of the branded systems developed by and for massive companies with large-scale needs don’t always translate to smaller teams and products. Every project has different requirements, so you should never assume that the best solution for InstaFace or MyPinBook is going to be the best solution for you.
您可能还会发现,由大型公司开发并为有大规模需求的大型公司开发的许多品牌系统并不总是转化为较小的团队和产品。 每个项目都有不同的要求,因此您永远不要以为InstaFace或MyPinBook的最佳解决方案将是您的最佳解决方案。
面向对象CSS(OOCSS) (Object-oriented CSS (OOCSS))
OOCSS is one of the original front-end architectures, and the initial inspiration for adding the @extend
directive to Sass. A project from Nicole Sullivan, it places a strong emphasis on finding the right granularity for CSS objects, a theme that comes up in most of the systems we’ll look at here.
OOCSS是原始的前端体系结构之一,也是将@extend
指令添加到Sass的最初灵感。 Nicole Sullivan的一个项目,着重强调为CSS对象找到合适的粒度 ,这是我们将在此处讨论的大多数系统中出现的主题。
Sullivan argues that rather than trying to match back-end objects, a CSS object should look for more granular design patterns that might be used across a variety of content types. A prime example is what she calls the media object—a fixed-size media element (such as an image or video) alongside fluid content such as text.
Sullivan认为,CSS对象应该尝试使用更细粒度的设计模式,这些模式可以用于多种内容类型,而不是尝试匹配后端对象。 一个典型的例子是她所说的媒体对象 -固定大小的媒体元素(例如图像或视频)以及诸如文本之类的流畅内容。
If you look at Facebook, which Sullivan helped refactor, you’ll see one media-object design used across the site to display a wide range of back-end objects—from stories and comments, to notifications, advertisements, and profile details. By defining objects at a granular level, a small amount of CSS can be used to style large swathes of the application.
如果您看一下由Sullivan协助重构的Facebook,您会发现在整个网站上使用了一种媒体对象设计来显示各种各样的后端对象,从故事和评论到通知,广告和个人资料详细信息。 通过在粒度级别上定义对象,可以使用少量CSS来对应用程序的大部分进行样式设置。
At its best, OOCSS is a powerful tool for simplifying CSS and perfecting the performance of large-scale applications. But taken to extremes, the OOCSS approach can leave you with a mess of single-purpose utility classes (such as .padding-left-10px
) that couple your HTML and CSS too tightly, and eliminate any maintainability you might get from more semantic code. You’ll have to find the right balance for each project.
最好的情况是,OOCSS是简化CSS和完善大型应用程序性能的强大工具。 但极端的是,OOCSS方法可能会给您带来一堆单一用途的实用程序类(例如.padding-left-10px
),这些类将您HTML和CSS紧密地结合在一起,并消除了您可能从更多语义代码中获得的可维护性。 您必须为每个项目找到合适的平衡。
Whatever else you do, the two main principles of OOCSS are worth keeping in mind (indeed, committing to memory) while you work out your own architecture:
无论您做什么,在设计自己的体系结构时,都应牢记OOCSS的两个主要原则(实际上是致力于内存):
Separate structure and skin. By having multiple design skins (colors, backgrounds, borders, and so on) that you can mix and match with structural objects, it’s possible to achieve more visual variety with less code. In practice, this also means decoupling styles from the base semantics of HTML tags. By styling classes (
.primary-header
) instead of tags (h2
), you have more flexibility to keep HTML meaningful, while applying consistent styles wherever they’re appropriate.结构与皮肤分开。 通过具有可以与结构对象混合和匹配的多个设计外观(颜色,背景,边框等),可以用更少的代码实现更多的视觉多样性。 实际上,这也意味着将样式与HTML标签的基本语义脱钩。 通过设置类(
.primary-header
)而不是标签(h2
)的样式,您可以更加灵活地使HTML有意义,同时在适当的地方应用一致的样式。Separate container and content. OOCSS objects should not be dependent on their location or context, but be reusable and able to fill whatever container they are given. This ensures that an object will look the same in any context, without developers having to guess what a given element or class will do in different situations.
容器和内容分开。 OOCSS对象不应该依赖于它们的位置或上下文,而是可以重用的,并且可以填充给定的任何容器。 这样可以确保对象在任何上下文中看起来都一样,而开发人员不必猜测给定元素或类在不同情况下会做什么。
There is no organizational structure built into OOCSS, but there is a framework available on GitHub that provides a number of common objects, as well as documentation on customizing the framework to your needs.
OOCSS中没有内置组织结构,但是GitHub上有一个框架 ,该框架提供了许多通用对象,以及有关根据需要定制框架的文档。
原子设计 (Atomic Design)
Atomic Design is also driven by questions of granularity. Initially devised by Brad Frost, an atomic project is broken down into five stages: atoms, molecules, organisms, templates, and pages. The idea is to style the stages in order, starting granular and working outwards, with each stage building on the one before.
原子设计也受粒度问题的驱动。 最初由Brad Frost设计的原子项目分为五个阶段:原子,分子,生物,模板和页面。 想法是按顺序对阶段进行样式设置,从粒状开始并向外进行,每个阶段都在之前的阶段构建。
According to Atomic Design, atoms can be abstract information such as color palettes, fonts, and typographic scales; they can also be default styles for tags such as form labels, buttons, and paragraphs. Since I can never remember the scientific terms, I break these two ideas down further and refer to the former as “configuration” or “settings” (having no output on their own), and the latter “base” or “initial” styles (having output).
根据原子设计, 原子可以是抽象信息,例如调色板,字体和印刷比例。 它们也可以是标签的默认样式,例如表单标签,按钮和段落。 由于我永远不记得科学术语,因此我将这两个想法进一步细分,将前者称为“配置”或“设置”(本身没有输出),而后者称为“基础”或“初始”样式(有输出)。
Atoms can be put together to form molecules. Combine an image with a paragraph and button (all atoms), and you have a simple product-listing molecule. Molecules are small components that do one task well. Group a number of these molecules together, and you have an organism (in this case, a gallery of products). Organisms are larger grouped components that form a section of the interface. Your site banner might also be an organism, combining a logo, navigation, and search form. I call these next two stages “patterns” and “components,” but it’s recommended that you work with your team to find terms you all understand clearly.
原子可以放在一起形成分子 。 将图像与段落和按钮(所有原子)结合起来,您将获得一个简单的产品列表分子。 分子是完成一项任务的小组件。 将许多这些分子归为一组,就可以得到一个有机体 (在这种情况下,是一个产品库)。 有机体是组成界面一部分的较大的分组组件。 您的网站横幅也可能是有机体,结合了徽标,导航和搜索表单。 我将接下来的两个阶段称为“模式”和“组件”,但是建议您与团队合作以找到大家都清楚理解的术语。
At this point, the developers of Atomic Design abandon their biochemical analogy, and move to templates. Templates combine the smaller molecules and organisms into actual layout structures. If you run a news site, you might have a list template and a detail template for your articles. Each specific instance of a template is called a page. The home page and archive page of your news site may both use the article-list template, but they have different content. Pages are the most specific combination of all the other stages.
此时,Atomic Design的开发人员放弃了他们的生化类比,转而使用模板 。 模板将较小的分子和有机体组合成实际的布局结构。 如果您运行新闻站点,则可能有文章的列表模板和详细信息模板。 模板的每个特定实例称为页面 。 新闻站点的主页和存档页面可能都使用文章列表模板,但是它们具有不同的内容。 页面是所有其他阶段的最具体组合。
A standard Atomic Design directory will be organized into these five stage-based folders:
标准的Atomic Design目录将组织到这五个基于阶段的文件夹中:
sass/
|
|– atoms/
| |– _colors.scss
| |– _buttons.scss
| …
|
|– molecules/
| |– _navigation.scss
| |– _search.scss
| …
|
|– organisms/
| |– _banner.scss
| |– _gallery.scss
| …
|
|– templates/
| |– _list.scss
| |– _detail.scss
| …
|
|– pages/
| |– _home.scss
| |– _archive.scss
| …
|
|- main.scss
Atomic Design also provides a framework called Pattern Lab. As with OOCSS, avoid confusing the framework with the design system philosophy. You can apply the philosophy anywhere, but the tools are still available if you need them. Frameworks can be a great way to keep code consistent across a large team or project, but always remember that you know your project better than Brad Frost, Nicole Sullivan, or the authors of this book. If there’s a conflict between your needs and the framework you’re using, always put your project first.
原子设计还提供了一个称为Pattern Lab的框架。 与OOCSS一样,避免将框架与设计系统哲学混淆。 您可以在任何地方应用该原理,但是如果需要,这些工具仍然可用。 框架可以是使大型团队或项目中的代码保持一致的一种好方法,但请始终记住,与Brad Frost,Nicole Sullivan或本书的作者相比,您对项目的了解要更好。 如果您的需求和使用的框架之间存在冲突,请始终将项目放在首位。
块,元素,修饰符(BEM) (Block, Element, Modifier (BEM))
BEM is a system developed by the Yandex team. This is a much more extensive system, with its fingers in every aspect of your code—from JSON data structures, to templates, as well as CSS.
BEM是Yandex团队开发的系统。 这是一个范围更广的系统,其手指遍及代码的各个方面-从JSON数据结构,模板到CSS。
The BEM CSS architecture is built around the three ideas in its title. Blocks are components of any size, and can be nested inside each other. The header
block might contain a logo
block, a navigation
block, and a search
block. Blocks are reusable, independent, and mobile—so they can be put anywhere on the page, and repeated as often as necessary. Elements are the constituent parts that belong to a specific block. A menu
block might be made up of four tab
elements. Modifiers are flags on blocks or elements that change their appearance, behavior, or state.
BEM CSS体系结构围绕其标题中的三个构想构建。 块是任何大小的组件,可以相互嵌套。 header
块可能包含logo
块, navigation
块和search
块。 块是可重用,独立且可移动的,因此可以将它们放置在页面上的任何位置,并根据需要重复进行。 元素是属于特定块的组成部分。 menu
块可能由四个tab
元素组成。 修饰符是更改其外观,行为或状态的块或元素上的标志。
The most immediately recognizable aspect of BEM syntax is an intricate naming convention that uses long class names instead of nesting selectors. Rather than targeting .block .element
, you would target .block__element
. There are variations on the exact syntax, but the formal documentation allow hyphens (-
) within a block, element, or modifier name; double underscore (__
) between block and element names; and single underscore (_
) before a boolean (true/false) modifier, or between a key-value modifier name and its given value.
BEM语法最容易识别的方面是复杂的命名约定,该约定使用长类名而不是嵌套选择器。 而不是定位.block .element
,而是定位.block__element
。 确切的语法有所不同,但是正式文档允许在块,元素或修饰符名称中使用连字符( -
)。 块和元素名称之间的双下划线( __
); 在布尔值(true / false)修饰符之前或键值修饰符名称与其给定值之间加一个下划线( _
)。
Here’s an example straight from the BEM documentation that defines a form
block with a _login
boolean modifier, a _theme_forest
key-value modifier, and two elements:
这是直接来自BEM文档的示例,该示例使用_login
布尔值修饰符, _theme_forest
键值修饰符和两个元素来定义form
块:
<form class="form form_login form_theme_forest">
<input class="form__input">
<input class="form__submit form__submit_disabled">
</form>
A related Sass partial would look like this:
相关的Sass部分看起来像这样:
.form {}
.form_theme_forest {}
.form_login {}
.form__input {}
.form__submit {}
.form__submit_disabled {}
When BEM naming became popular, people started using the Sass parent selector (&
) to automatically generate their BEM class names with less repetition in the code:
当BEM命名变得流行时,人们开始使用Sass父选择器( &
)自动生成其BEM类名,并在代码中减少重复:
.form {
border: 1px solid black;
&__submit {
background-color: green;
&_disabled {
background-color: gray;
}
}
}
.form {
border: 1px solid black;
}
.form__submit {
background-color: green;
}
.form__submit_disabled {
background-color: gray;
}
On the surface, this works great—but it comes at the cost of searchability. If another developer has to find the .form__submit_disabled
Sass in order to make a change, searching your Sass files for .form__submit_disabled
will fail to return any results.
从表面上看,这很好用,但是却以可搜索性为代价。 如果另一个开发人员必须找到.form__submit_disabled
Sass才能进行更改,则在Sass文件中搜索.form__submit_disabled
将无法返回任何结果。
The BEM file structure goes beyond CSS and Sass, organizing all assets (JavaScript, CSS, images, and so on) into shared directories by block. Elements and modifiers have their own subdirectories using the same underscore-driven naming conventions:
BEM文件结构超出了CSS和Sass的范围,将所有资产(JavaScript,CSS,图像等)按块组织到共享目录中。 元素和修饰符具有自己的子目录,使用相同的下划线驱动命名约定:
blocks/
|- input/
| |- _type/
| | |- input_type_search.css
| |
| |- __box/
| | |- input__box.css
| |
| |- input.css
| |- input.js
|
|- button/
| |- button.css
| |- button.js
| |- button.png
CSS的可扩展和模块化架构(SMACSS) (Scalable and Modular Architecture for CSS (SMACSS))
SMACSS is a book, workshop, and philosophy by Jonathan Snook. Like Atomic Design, this architecture uses five categories for organizing your CSS, except that they aren’t organized from small to large. Detailed naming patterns are provided to help keep class names consistent. It’s one of the most popular brand-name architectures, and may even be the most comprehensive.
SMACSS是Jonathan Snook的书,讲习班和哲学。 像Atomic Design一样,此体系结构使用五类来组织CSS,不同之处在于它们不是按照大小排列的。 提供了详细的命名模式以帮助保持类名的一致性。 它是最受欢迎的品牌架构之一,甚至可能是最全面的。
The five categories here are base, layout, module, state, and theme. Base rules define the default style of elements, which work similarly to the atoms of Atomic Design. Layout styles are used to break the document into sections that can contain modules, the individual components of a design. State rules define different JavaScript-dependent states for a module or layout; that is, how does it change when it is active or inactive, collapsed or expanded? Most sites have no need for themes, but they can be used to describe multiple style options for the same modules.
这里的五个类别是基础,布局,模块,状态和主题。 基本规则定义了元素的默认样式,这些元素的工作方式类似于“原子设计”的原子。 布局样式用于将文档分为可包含模块 (设计的各个组件)的部分。 状态规则为模块或布局定义了不同JavaScript相关状态; 也就是说,当它处于活动状态或非活动状态,崩溃或扩展时,它将如何变化? 大多数站点不需要主题 ,但是它们可以用于描述同一模块的多个样式选项。
In order to help keep CSS and HTML modules small and mobile, SMACSS pays special attention to what Snook calls the depth of applicability. You may know of the Sass “inception rule,” which states that you should never nest selectors more than three layers deep. That rule helps to keep selectors short (no more than three layers), but the depth of applicability is a bit different. Rather than counting the number of layers, it counts the total DOM distance between the first and last layers.
为了使CSS和HTML模块保持小巧和可移动性,SMACSS特别注意了Snook所说的适用性 。 您可能知道Sass的“初始规则”,该规则指出,嵌套选择器的深度不得超过三层。 该规则有助于使选择器简短(不超过三层),但是适用性的深度有所不同。 它不计算层数,而是计算第一层和最后一层之间的总DOM距离 。
Let’s look at a simple example. Since .mammalia > .primates > .hominidae > .sapiens > .rollsman > .erin
has a depth of six, the same basic selector written as .mammalia .sapiens .erin
would still have a depth of six. By shortening the selector, we’ve lowered the specificity (a good thing!), but we still have a large depth of applicability. The problem with so much depth is that it makes our CSS more dependent on a particular HTML structure. This is generally solved by keeping our HTML and CSS components small and independent from their containers.
让我们看一个简单的例子。 由于.mammalia > .primates > .hominidae > .sapiens > .rollsman > .erin
的深度为6,因此写为.mammalia .sapiens .erin
的相同基本选择器的深度仍为6。 通过缩短选择器,我们降低了特异性(这是一件好事!),但适用范围仍然很广。 如此深入的问题在于,它使我们CSS更加依赖于特定HTML结构。 通常可以通过使我们HTML和CSS组件保持较小且独立于其容器来解决此问题。
雨果7-1 (Hugo’s 7-1)
Hugo uses a variation of SMACSS for organizing Sass partials. He calls it the “7-1” system, because it uses seven folders of partials and one master file to pull them all together.
雨果(Hugo)使用SMACSS的变体来组织Sass部分。 他称其为“ 7-1 ”系统,因为该系统使用了七个部分文件夹和一个主文件来将它们全部拉在一起。
The base/ folder contains broad standards across a site—such as a reset, default styles for common HTML tags, common animations, and basic typography. The layout folder includes everything one might need for laying out the structure of a site; for example, boilerplate-like headers, footers, and navigation, as well as your grid system and layout helpers. The components folder is organized into partials by component; the pages folder contains any page-specific styles; and a themes
folder holds any theme-related styles (if your project has multiple themes).
base /文件夹包含整个站点的广泛标准,例如重置,常见HTML标签的默认样式,常见动画和基本排版。 布局文件夹包含布局网站结构可能需要的所有内容; 例如,样板样的页眉,页脚和导航,以及网格系统和布局助手。 组件文件夹按组件分为多个部分。 pages文件夹包含任何特定于页面的样式; 一个themes
文件夹包含任何与主题相关的样式(如果您的项目有多个主题)。
7-1 also includes an abstracts folder for Sass tools and helpers, which is organized into partials for global variables, functions, mixins, and placeholders. Nothing in this folder should output any CSS if compiled on its own.
7-1还包括一个用于Sass工具和助手的abstracts文件夹,该文件夹被组织为局部变量,用于全局变量,函数,mixin和占位符。 如果自行编译,此文件夹中的任何内容均不应输出任何CSS。
Hugo leaves the possibility of organizing these partials by topic (typography, colors, etc.) rather than type (variables, mixins, functions) for larger projects, but I recommend that across the board. The topic is always the more important distinction in my mind. Placeholders are the only type that I treat in any special way, because their output remains in the location they are defined—while variables, functions, and mixins create output where they are used.
对于较大的项目,Hugo保留了按主题(印刷术,颜色等)而不是类型(变量,mixin,函数)组织这些部分的可能性,但我还是建议您全面使用。 在我看来,主题始终是最重要的区别。 占位符是我以任何特殊方式处理的唯一类型,因为占位符的输出保留在它们定义的位置,而变量,函数和mixins在使用它们的位置创建输出。
Finally, there is a vendors folder for third-party libraries, frameworks, and toolkits such as Normalize, Bootstrap, jQueryUI, FancyButtonsOMG, and so on. These are often kept separate so as to not edit them should they need upgrading later.
最后,还有一个供应商的第三方库,框架,以及诸如正常化,引导,jQueryUI的,FancyButtonsOMG等工具包文件夹中。 它们通常保持分开,以便以后需要升级时不进行编辑。
Put it all together, and you have a Sass directory similar to this:
放在一起,您将拥有一个类似于以下内容的Sass目录:
sass/
|
|– base/
| |– _reset.scss # Reset/normalize
| |– _typography.scss # Typography rules
| … # Etc.
|
|– components/
| |– _buttons.scss # Buttons
| |– _carousel.scss # Carousel
| |– _cover.scss # Cover
| |– _dropdown.scss # Dropdown
| … # Etc.
|
|– layout/
| |– _navigation.scss # Navigation
| |– _grid.scss # Grid system
| |– _header.scss # Header
| |– _footer.scss # Footer
| … # Etc.
|
|– pages/
| |– _home.scss # Home specific styles
| |– _contact.scss # Contact specific styles
| … # Etc.
|
|– themes/
| |– _theme.scss # Default theme
| |– _admin.scss # Admin theme
| … # Etc.
|
|– utils/
| |– _variables.scss # Sass Variables
| |– _functions.scss # Sass Functions
| |– _mixins.scss # Sass Mixins
| |– _helpers.scss # Class & placeholders helpers
|
|– vendors/
| |– _bootstrap.scss # Bootstrap
| |– _jquery-ui.scss # jQuery UI
| … # Etc.
|
`– main.scss # Main Sass file
倒三角形CSS(ITCSS) (Inverted Triangle CSS (ITCSS))
ITCSS is a new architecture that is just starting to gain attention. This system from Harry Roberts does a great job defining the problem of CSS architecture and proposing a solution that comes directly out of the CSS language. Rather than working around inheritance and specificity, Roberts puts them at the center of his methodology.
ITCSS是一个刚刚开始引起关注的新体系结构。 哈里·罗伯茨 ( Harry Roberts)的这个系统在定义CSS体系结构问题和提出直接来自CSS语言的解决方案方面做得很好。 罗伯茨没有围绕继承和特殊性开展工作,而是将它们置于他的方法论的中心。
ITCSS organizes all your Sass and CSS based on three metrics: reach, specificity, and explicitness—visualized as an inverted triangle, as shown below:
ITCSS根据三个指标来组织您的所有Sass和CSS:覆盖率,特异性和明确性-可视为倒三角形,如下所示:
Code should be organized from least to most explicit, starting with general catch-all rules (such as a reset) and moving up to more explicit styles (such as .contact-form
). Similarly, code is organized from broadest to narrowest reach—so that styles affecting more HTML come early in the code, and styles with a more localized application come later. Finally, code is organized from lowest to highest specificity, so that later code can always override earlier code.
代码应从最小到最明确地组织,从通用的总体规则(例如重置)开始,然后发展到更明确的样式(例如.contact-form
)。 同样,代码是从最广泛的范围到最窄的范围进行组织的,因此影响更多HTML的样式会出现在代码的早期,而本地化的应用程序的样式会在稍后出现。 最后,代码是按照从最低到最高的特异性组织的,因此以后的代码可以始终覆盖以前的代码。
With those metrics in mind, the triangle is broken down into seven layers. Each layer is more specific, explicit, and narrow-reaching than the layer before it, as shown here:
考虑到这些指标,三角形被分为七个层。 每一层都比它之前的层更加具体,明确和狭窄,如下所示:
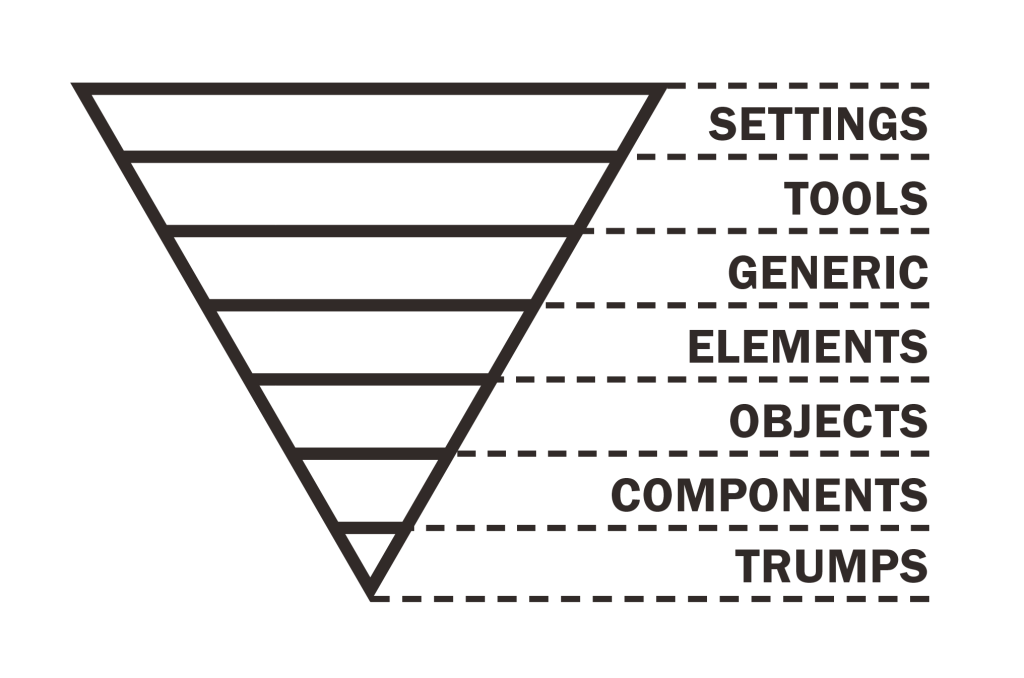
Figure 9.3. ITCSS’s layers
图9.3。 ITCSS的层
Let’s explore what these layers are in detail. Settings contains global Sass configuration that can be accessed anywhere in the project, such as font sizes, colors, and other project configuration. Tools are global functions and mixins that are helpful across the project and not specific to one component. Generic is the first layer with CSS output of its own, which includes browser resets or normalization, global box-sizing, and any other broad-scoped rules. The elements layer provides default styles for bare HTML elements such as links and paragraphs. It’s similar to the generic layer, except that it provides a more opinionated style.
让我们探究这些层的细节。 设置包含可以在项目中的任何位置访问的全局Sass配置,例如字体大小,颜色和其他项目配置。 工具是全局功能和混入工具 ,它们在整个项目中很有用,而并非特定于一个组件。 通用是具有自己CSS输出的第一层,其中包括浏览器重置或规范化,全局框大小调整以及任何其他广义规则。 elements层为裸HTML元素(例如链接和段落)提供默认样式。 它与通用层相似,不同之处在于它提供了更个性化的样式。
ITCSS objects are similar to OOCSS objects, and are defined in class-based selectors. They define reusable patterns that have a consistent structure no matter what content or cosmetic style is applied, just like the OOCSS media object does. Components are recognizable pieces of an interface, such as a contact form or a product listing. After the initial setup, this is where the majority of a project’s feature-building work takes place. Finally, trump styles can be used to override any other layer. Trumps should be used sparingly, and have as narrow a scope as possible.
ITCSS 对象类似于OOCSS对象,并且在基于类的选择器中定义。 它们定义了可重复使用的模式,无论应用何种内容或修饰样式,其结构都保持一致,就像OOCSS媒体对象一样。 组件是界面的可识别部分,例如联系表或产品列表。 初始设置后,这里就是项目大部分功能构建工作的地方。 最后, 王牌样式可用于覆盖任何其他图层。 特朗普应谨慎使用,范围应尽可能狭窄。
All these layers can be organized into groups of partials. Roberts uses a multilevel file-naming convention (layer-name.partial-name.scss
), but we’d recommend using folders instead. The results could look like this:
所有这些层都可以组织成部分局部。 Roberts使用了多层文件命名约定( layer-name.partial-name.scss
),但是我们建议使用文件夹代替。 结果可能如下所示:
@import "settings/global";
@import "settings/colors";
@import "tools/functions";
@import "tools/mixins";
@import "generic/box-sizing";
@import "generic/normalize";
@import "elements/headings";
@import "elements/links";
@import "objects/wrappers";
@import "objects/grid";
@import "components/site-nav";
@import "components/buttons";
@import "components/carousel";
@import "trumps/clearfix";
@import "trumps/utilities";
@import "trumps/ie8";
Miriam的Mix-n-Match (Miriam’s Mix-n-Match)
All that is well and good, but I’m writing this chapter and I think my own architecture is way cooler than anything else we’ve discussed. I’m yet to give it a name, but I will as soon as I decide to tour the universe giving workshops to all my adoring fans. A girl can dream, right?
一切都很好,但是我正在写这一章,我认为我自己的体系结构比我们讨论过的其他任何事物都要酷。 我还没有给它起个名字,但是我一决定去宇宙巡回演出,为所有我的崇拜者们准备工作坊,我就给它起个名字。 一个女孩可以做梦,对吗?
To tell you the truth, I love parts of all these systems—especially ITCSS. I take what works for my team, and make adjustments as needed from one project to the next. For me, it all starts with one rule: follow the cascade. In practice, it looks a lot like ITCSS or Atomic Design (though I find the latter’s biochemical metaphor confusing). I use the same metrics, but break down the categories in slightly different ways.
实话实说,我喜欢所有这些系统的一部分,尤其是ITCSS。 我选择适合自己团队的方法,并根据需要对一个项目进行调整。 对我而言,一切都从一条规则开始:遵循层叠。 实际上,它看起来很像ITCSS或Atomic Design(尽管我发现后者的生化隐喻令人困惑)。 我使用相同的指标,但以略有不同的方式细分类别。
I start with Sass config files that have no output but define all the parameters of a design: colors, fonts, sizes, media-queries, z-indexes, and so on. In my case, it’s almost entirely Sass map variables accessed with a powerful set of functions and mixins I take from project to project: OddBird’s Accoutrement toolkits. Chris Sauvé refers to this approach as a “Sass Central Nervous System”—a consistent system for maintaining and accessing abstract meta-patterns and style guidelines. Ours look something like this:
我从Sass配置文件开始,该文件没有输出,但定义了设计的所有参数:颜色,字体,大小,媒体查询,z索引等。 就我而言,几乎所有的Sass映射变量都可以通过一组功能强大的函数和mixin访问,这些功能和混合我从一个项目到另一个项目: OddBird的Accoutrement工具包。 克里斯·索维(ChrisSauvé)将此方法称为“ Sass中枢神经系统” —一种用于维护和访问抽象元模式和样式准则的一致系统。 我们看起来像这样:
// Accoutrement Config
// -------------------
$colors: (
// base color palette
'brand-blue': hsl(195, 100%, 43%),
'brand-red': hsl(0, 100%, 50%),
'brand-pink': hsl(330, 100%, 45%),
// color style guide
'background': hsl(0, 0%, 100%),
'text': 'brand-blue' ('shade': 80%),
'action': 'brand-pink',
'focus': 'brand-blue',
);
$sizes: (
// base font size
'body-text': 22px,
// type sizes
'rhythm': 'body-text' ('minor-third': 2),
'h1': 'body-text' ('minor-third': 3),
'h2': 'body-text' ('minor-third': 2),
'h3': 'body-text' ('minor-third': 1),
// other
'corners': 3px,
'page': 30rem,
);
$fonts: (
// hosted web font
'body': (
'name': 'CenturyOldStyle',
'stack': ('Baskerville', 'Palatino', 'Cambria', 'Georgia', 'serif'),
'regular': 'CenturyOldStyle-regular', // webfont file names...
'italic': 'CenturyOldStyle-italic',
'bold': 'CenturyOldStyle-bold',
),
// web-safe font stack
'code': (
'name': 'Consolas',
'stack': ('Menlo', 'Monaco', 'Lucida Console', 'Liberation Mono', 'DejaVu Sans Mono', 'Bitstream Vera Sans Mono', 'Courier New', 'monospace', 'serif')
),
);
The toolkit layer is prebuilt, and moves with us from project to project. It includes functions and mixins that put our configuration to work: automating @font-face
imports, font-stacks, and typographical rhythms, as well as applying our color palette. It also helps with accessible color contrasts, and automatically generates a visual style guide, so we can see the fonts, colors, and sizes in action.
工具箱层是预先构建的,并且随我们在项目之间移动。 它包含使我们的配置生效的功能和混合函数:自动@font-face
导入,字体堆栈和印刷节奏,以及应用我们的调色板。 它还有助于获得可访问的色彩对比,并自动生成视觉样式指南,因此我们可以查看实际使用的字体,颜色和大小。
The next level up is what I call initial styles—resets, web font imports, global defaults, and so on. This is the first layer of code with actual CSS output, and it’s a thin layer. At this point we’re not styling any real patterns, just trying to establish a slightly more beautiful and branded version of the browser defaults.
下一个升级就是我所说的初始样式-重置,Web字体导入,全局默认设置,等等。 这是具有实际CSS输出的第一层代码,并且是一薄层。 在这一点上,我们还没有设置任何实际的样式,只是尝试建立一个稍微更漂亮,更品牌化的浏览器默认设置。
From there I often establish the site layout, adding patterns as needed. The layout partials are similar to Hugo’s, describing all the primary structures of the site. Patterns are design objects, similar to objects in OOCSS and ITCSS. They’re not related to specific content, and might be used anywhere, for anything. For example, buttons and form elements are always some of my first design patterns on a project.
我经常从那里建立站点布局 ,并根据需要添加模式。 布局局部与Hugo相似,描述了站点的所有主要结构。 模式是设计对象,类似于OOCSS和ITCSS中的对象。 它们与特定的内容无关,可以在任何地方用于任何事物。 例如,按钮和表单元素始终是我在项目中的第一个设计模式。
Patterns are abstract, and have no real meaning until they’re used in a component—the actual bits of user interface that appear on a site. Components should follow all the rules described earlier in the chapter: reusable, repeatable, and able to fit in any container. What others systems call page and theme styles are usually defined either as layout templates or components that just happen to be full screen. Any vendor code that I use will come through a packaging system such as npm, and live outside my visible Sass directory:
模式是抽象的,在将它们用于组件之前是没有实际意义的, 组件是出现在站点上的用户界面的实际部分 。 组件应遵循本章前面所述的所有规则:可重用,可重复并且能够放入任何容器中。 其他系统所称呼的页面和主题样式通常定义为布局模板或恰好是全屏的组件。 我使用的任何供应商代码都将通过npm之类的打包系统提供,并位于我可见的Sass目录之外:
sass/
|
|– config/
| |– _colors.scss # Color palettes
| |– _fonts.scss # Font palettes
| … # Etc.
|
|– initial/
| |– _init.scss # reset/normalization
| |– _root.scss # global defaults (mostly :root, html, body)
| |– _webfonts.scss # @font-face imports
| … # Etc.
|
|– layout/
| |– _navigation.scss # Navigation
| |– _banner.scss # Site Banner
| … # Etc.
|
|– patterns/
| |– _buttons.scss # Buttons
| |– _dropdown.scss # Dropdown
| … # Etc.
|
|– components/
| |– _calendar.scss # Calendar widget styles
| |– _contact.scss # Contact form styles
| … # Etc.
|
|- main.scss # The primary Sass file to be compiled
Lately, I’ve also included a styleguide folder, and an extra styleguide.scss
root Sass file to be compiled separately. These files contain any styleguide-specific components not required by the main app—styles for the color palette, font specimens, and so on.
最近,我还包括了一个styleguide文件夹,以及一个额外的styleguide.scss
根Sass文件,可以分别进行编译。 这些文件包含主应用程序不需要的任何特定于样式指南的组件,例如调色板的样式,字体样本等。
Sass中的模块化导入4 (Modular Imports in Sass 4)
As this chapter was being written, the core Sass designers, Natalie Weizenbaum and Chris Eppstein, were working out the details for modular imports, the major new feature that is driving plans for Sass 4. The specifics are still in flux, but the direction they’re going in is exciting, so it’s worth giving you a sneak peak at what they’ve done so far.
在撰写本章时,Sass的核心设计师Natalie Weizenbaum和Chris Eppstein正在研究模块化导入的细节,这是推动Sass 4计划的主要新功能。具体细节仍在不断变化,但是他们的方向是不断变化的进来很令人兴奋,因此值得您一窥他们到目前为止所做的事情。
Modular imports are a move away from the CSS @import
syntax towards one that is more powerful and Sass-specific. Where Sass imports currently work as though the entire imported document has been cut and pasted into place, modular imports provide a lot more control for the developer—inspired by best practice in languages such as Python and Dart. It will probably look a little like this:
模块化导入是从CSS @import
语法向更强大且特定于Sass的语法的转变。 在Sass导入当前可以正常工作,就好像整个导入文档都被剪切并粘贴到位的情况一样,模块化导入为开发人员提供了更多控制权-受Python和Dart等语言的最佳实践的启发。 它可能看起来像这样:
@use 'path/to/sitepoint/author' as 'miriam';
.sitepoint {
@include miriam.write('Jump Start Sass');
-webkit-paycheck: miriam.money('millions');
}
Okay, there may not be a -webkit-paycheck
property coming anytime soon, but the rest looks good. So what’s it all about, and why do we need it?
好的,很快就不会有-webkit-paycheck
属性了,但是其余的看起来不错。 那么这是怎么回事,为什么我们需要它?
地区性 (Locality)
With the current Sass import system, variables, mixins, and functions live in a global namespace across all files; conflicts are common. It’s impossible to tell by looking at a single Sass file what already exists in that global space; however, with modular imports, nothing is made global unless I explicitly request it. The @use
directive will be visible at the top of any importing file, giving me a complete list of available APIs and the power to namespace each however I see fit.
在当前的Sass导入系统中,变量,混合和函数都存在于所有文件的全局命名空间中。 冲突很普遍。 通过查看单个Sass文件无法判断该全局空间中已经存在的内容。 但是,对于模块化导入,除非我明确要求,否则什么都不会成为全局的。 @use
指令将在任何导入文件的顶部可见,从而为我提供了可用API的完整列表,并为我提供了合适的命名空间。
If you @use 'example/grids' as 'grid'
at the top of a file, and the example/grids.scss file contains a span()
mixin and a gutter()
function, then they become available in your file as grid.span()
and grid.gutter()
(the .
syntax is still under discussion). The same will be possible with variables, so a $columns
variable would be available to as $grid.columns
.
如果@use 'example/grids' as 'grid'
在文件顶部使用@use 'example/grids' as 'grid'
,并且example / grids.scss文件包含span()
mixin和gutter gutter()
函数,则它们将在您的文件中作为grid.span()
可用grid.span()
和grid.gutter()
( .
语法仍在讨论中)。 变量也可能如此,因此$columns
变量可作为$grid.columns
。
// example/grids.scss
@mixin span(…) { … }
@function gutter(…) { … }
$columns: 12;
// my-file.scss
@use 'example/grids' as 'grid';
.column {
@include grid.span(5 of $grid.columns);
margin-bottom: grid.gutter();
}
Sass will default to using the filename as a prefix if none is provided, and also allow you to remove the prefix when you need to. It’s still not clear if prefixing will work with placeholder selectors.
如果未提供文件名,Sass将默认使用文件名作为前缀,并且还允许您在需要时删除该前缀。 前缀是否可以与占位符选择器一起使用还不清楚。
In addition to using a file with or without a given prefix, it might be possible to use an entire file as a mixin, so you can apply the code of that file anywhere you want—even in a nested context. The syntax is still under consideration, but it would make the entire CSS contents (that are not wrapped in a mixin) available to you as a single mixin.
除了使用带有或不带有给定前缀的文件之外,还可以将整个文件用作mixin ,因此即使在嵌套上下文中,也可以在任何位置应用该文件的代码。 语法仍在考虑中,但是它将使整个CSS内容(未包装在mixin中)可以作为单个mixin使用。
封装形式 (Encapsulation)
Modular imports will also give developers—especially library authors—more power over their public API. Currently, when you load a Sass library such as Susy, you gain access to pages and pages of undocumented functions that you’ll never use. I’ve done my best to hide those functions behind long names like _susy-valid-column-math
, but they still clutter the global namespace unnecessarily. With encapsulation, you’ll have control over which mixins, functions, variables, and (possibly) placeholders should be made public. Adding -
or _
to the start of a name will define it as private.
模块化导入还将为开发人员(尤其是库作者)提供更多使用其公共API的功能。 当前,当您加载诸如Susy之类的Sass库时,您将获得对您将永远不会使用的页面和未记录功能的页面的访问。 我已经尽力将那些函数隐藏在诸如_susy-valid-column-math
类的长名称后面,但是它们仍然会不必要地使全局名称空间混乱。 使用封装,您可以控制应公开哪些混合,函数,变量和(可能)占位符。 在名称的开头添加-
或_
会将其定义为私有。
There is also talk of a @forward
directive that would allow authors to pass the API from one module along as part of another. If you wanted to build a Susy flexbox extension, for example, you could tell your extension to forward the Susy API along to your users.
还讨论了@forward
指令,该指令允许作者将一个模块的API作为另一个模块的一部分传递。 例如,如果要构建Susy flexbox扩展,可以告诉扩展将Susy API转发给用户。
All of this, of course, is still in the works, and likely to change before it becomes available later in the year. I can’t wait to see how it turns out—in what ways it changes Sass architecture, and helps the Sass ecosystem.
当然,所有这些仍在进行中,并且有可能在今年晚些时候面世之前进行更改。 我等不及要看结果了,它以什么方式改变了Sass的体系结构,并帮助了Sass生态系统。
包装东西 (Wrapping Things Up)
We’ve taken a fairly in-depth look at architecture for your Sass projects. We started off by discussing @import
, and seeing how you can use it to split your project code into small logical units and organizing it across multiple files, partials and folders. This forms the basis of any projects architecture. We then moved on to discuss a whole range of architecture options; which you choose will depend on your own projects and preferences. Finally we looked at future options for modular imports that should be in Sass 4.
我们已经对Sass项目的体系结构进行了相当深入的研究。 我们首先讨论@import
,然后看看如何使用它将项目代码拆分为较小的逻辑单元,并在多个文件,局部文件和文件夹中进行组织。 这构成了任何项目体系结构的基础。 然后,我们继续讨论整个体系结构选项。 选择哪种取决于您自己的项目和偏好。 最后,我们研究了应该在Sass 4中使用的模块化导入的未来选项。
翻译自: https://www.sitepoint.com/architecture-in-sass/
如若内容造成侵权/违法违规/事实不符,请联系编程学习网邮箱:809451989@qq.com进行投诉反馈,一经查实,立即删除!
相关文章
- 深度学习在计算机视觉领域图像应用总结
简单的回顾的话,2006年Geoffrey Hinton的论文点燃了“这把火”,现在已经有不少人开始泼“冷水”了,主要是AI泡沫太大,而且深度学习不是包治百病的药方。 计算机视觉不是深度学习最早看到突破的领域,真正让大家大吃一惊…...
2024/5/5 19:28:31 - 跌倒检测_使用姿势估计的跌倒检测
跌倒检测Fall detection has become an important stepping stone in the research of action recognition — which is to train an AI to classify general actions such as walking and sitting down. What humans interpret as an obvious action of a person falling face…...
2024/5/5 18:25:28 - 推荐!最适合初学者的18个经典开源计算机视觉项目
英语原文:18 All-Time Classic Open Source Computer Vision Projects for Beginners 翻译:雷锋字幕组(小哲) 概述 开源计算机视觉项目是在深度学习领域中获得一席之地的绝佳路径 开始学习这18个非常受欢迎的经典开源计算机视觉…...
2024/4/20 20:47:05 - AI 综述专栏 | 超长综述让你走近深度人脸识别
来源:人工智能前沿讲习班 作者: 葛政相信做机器学习或深度学习的同学们回家总会有这样一个烦恼:亲朋好友询问你从事什么工作的时候,如何通俗地解释能避免尴尬?我尝试过很多名词来形容自己的工作:机器学习&…...
2024/5/5 18:09:56 - deepfakes超进化!反复变脸行云流水,完全没有PS痕迹,推特10万点赞
鱼羊 栗子 发自 凹非寺 量子位 报道 | 公众号 QbitAIDeepfakes要冲出天际了。这里有一段神奇的视频 (被我裁成了动图) ,请擦亮眼睛观看。因为讲话的人类,中途从比尔哈德,变成了施瓦辛格:行云流水。说不清什么时候,已经…...
2024/4/20 20:47:02 - 深度人脸表情识别技术综述,没有比这更全的了
策划 & 编辑 | Natalie编译 | 马卓奇AI 前线导读: 面部表情识别技术(FER)正逐渐从实验室数据集测试走向挑战真实场景下的识别。随着深度学习技术在各领域中的成功,深度神经网络被越来越多地用于学习判别性特征表示。目前的深度…...
2024/4/20 20:47:01 - 【总结】最全1.5万字长文解读7大方向人脸数据集v2.0版,搞计算机视觉怎能不懂人脸...
人脸图像是计算机视觉领域中研究历史最久,也是应用最广泛的图像。从人脸检测、人脸识别、人脸的年龄表情等属性识别,到人脸的三维重建等,都有非常多的数据集被不断整理提出,极大地促进了该领域的发展。本次,我们从人脸…...
2024/5/5 17:42:01 - 【VGG16】口罩人脸分类(动态图版)
文章目录问题导入一、基本概念1. 动态图DyGraph2. VGG模型二、数据集简介三、实验步骤0. 导入模块1. 数据准备2. 网络配置3. 模型训练4. 模型评估5. 模型预测写在最后问题导入 2020年上半年,新冠病毒在全球肆虐,武汉大学率先公开了口罩遮挡人脸数据集&a…...
2024/4/21 1:17:01 - tensorflow显存不足报错CUBLAS_STATUS_ALLOC_FAILED解决
tensorflow显存不足报错CUBLAS_STATUS_ALLOC_FAILED解决 TensorFlow执行,报显存不足错误,如下 解决方法 通过设定config为使用的显存按需自动增长,避免显存被耗尽,可进行有效的预防显存不足问题。 # 定义TensorFlow配置 conf…...
2024/5/5 21:58:27 - deep face 换脸功能 探索
deepfake刚出来的时候超级火,最近终于有大佬出资源找我做这块,上手开GAN。 先从GitHub上下代码。 配置环境,Ubuntu一激动装了18,折腾好几天各种坑,乖乖回到了16,一天搞定所有环境。 机器配置要求有n卡&a…...
2024/5/5 17:23:41 - 滴滴云RTX2080ti尝鲜,云端游戏卡搞起!
游戏显卡中RTX2080Ti可以说是业界翘楚了,虽然不是专门为深度学习而设计,但是在深度学习上的性能表现也是不错滴。而云端深度学习卡一般都是以Tesla系列为主,虽然号称专业的深度学习卡,但是某些型号也是很渣。 不知道有没有人想过把…...
2024/5/5 18:00:11 - Win10显卡跑不满?是时候给系统洗洗澡了,换系统提高GPU利用率
有些模型用Win7或Win8跑的好好的,换成Win10以后就跑不动了?这是个什么问题呢,经过查询发现的确是Windows10系统的锅,请看:Win10 CUDA效率问题.也就是说Win10系统会默认预留20%的显存,而这个问题从Win10发布…...
2024/5/5 12:27:37 - AI黑科技:目前最流行的人工智能换脸软件(FakeAPP/Faceswap/Openfaceswap/Deepfacelab)的简介、对比之详细攻略
AI黑科技:目前最流行的人工智能换脸软件(FakeAPP/Faceswap/Openfaceswap/Deepfacelab)的简介、对比之详细攻略 目录 目前最流行的人工智能换脸软件的简介 FakeAPP 1、简介 2、使用 Faceswap...
2024/4/21 1:16:57 - Quick96, SAEHD重置 DeepFaceLab更新至2019.12.28
如标题一样,这是一个很不幸的消息,Quick96,SAEHD重设了,你的所谓的丹或许已经无法再用。所以看到下图不必惊慌,这是正常的,因为SAE也经过多次重置,不经历重置的模型不是成熟的模型(笑…...
2024/5/4 3:35:20 - GTX 750等低配显卡如何玩转Deepfakes?
这里说的Deepfakes软件还是DeepFaceLab,人工智能换脸,是使用深度学习方法来实现的。而深度学习程序对电脑配置要求是非常高的,尤其是跑模型这个环节。很多低配电脑,根本就跑步起来。比如像GTX 750 ,1G显存。 默认情况…...
2024/4/21 1:16:54 - 比脸软件测试自学,【AI测试学习】FakeApp, Faceswap, DeepFaceLab等Deepfakes换脸程序的简单对比 (转)...
目前用于深度换脸的程序基本都是用python编程语言基于tensorflow进行计算。以下列出几款常用的换脸程序优缺点浅析,用户可以根据自己的爱好和水平来选择,以下软件均需要先安装windows 版本的 VS2015,CUDA9.0和CuDNN7.0.5下面几个程序的对比和官网下载地址…...
2024/5/5 21:41:13 - 简单介绍SimSwap(类似DeepFaceLab)单张图视频换脸的项目
DeepFaceLab相关文章 一:《简单介绍DeepFaceLab(DeepFake)的使用以及容易被忽略的事项》 二:《继续聊聊DeepFaceLab(DeepFake)不断演进的2.0版本》 三:《如何翻译DeepFaceLab(DeepF…...
2024/5/5 3:29:28 - DeepFaceLab报错,integer division or modulo by zero
DeepFaceLab的集成环境在众多换脸软件中是做的最好的。但是使用过程也会出现一些错误,主要的错误有两个,一个是你配置太低OOM了,主要体现显存太低。第二个是版本不对应。比如你原先用的cuda9.0或cuda9.2, 然后你升级到了Deepfacelab10.1&…...
2024/4/21 1:16:58 - DeepFake技术--fakeapp, faceswap, deepfacelab等deepfakes换脸程序的简单对比
目前用于深度换脸的程序基本都是用python编程语言基于tensorflow进行计算。以下列出几款常用的换脸程序优缺点浅析,用户可以根据自己的爱好和水平来选择,以下软件均需要先安装windows 版本的 VS2015,CUDA9.0和CuDNN7.0.5 下面几个程序的对比和官网下载地…...
2024/4/21 1:16:50 - DeepFaceLab进阶(4):通过Colab免费使用Tesla K80 跑模型!
当学会了换脸软件DeepFaceLab基本使用,各种参数配置,各有优化技能之后。唯一约束你的可能是电脑配置。 CPU能跑,但是慢到怀疑人生,低配模型都得跑一周 低配显卡,显存不够,H128 根本就跑不起来,…...
2024/4/21 1:16:49
最新文章
- 车载诊断技术 --- Service 22读取DID怎么会导致ECU不在线
车载诊断技术 — Service 22读取DID怎么会导致ECU不在线 我是穿拖鞋的汉子,魔都中坚持长期主义的汽车电子工程师。 老规矩,分享一段喜欢的文字,避免自己成为高知识低文化的工程师: 屏蔽力是信息过载时代一个人的特殊竞争力,任何消耗你的人和事,多看一眼都是你的不对。非…...
2024/5/5 22:29:43 - 梯度消失和梯度爆炸的一些处理方法
在这里是记录一下梯度消失或梯度爆炸的一些处理技巧。全当学习总结了如有错误还请留言,在此感激不尽。 权重和梯度的更新公式如下: w w − η ⋅ ∇ w w w - \eta \cdot \nabla w ww−η⋅∇w 个人通俗的理解梯度消失就是网络模型在反向求导的时候出…...
2024/3/20 10:50:27 - 【开源语音项目OpenVoice](一)——实操演示
目录 一、前菜 1、Python选择 2、pip源切换 3、ffmpeg配置问题 4、VSCode添加Jupyter扩展 二、配置虚拟环境 1、下载源码 方法一 直接下载源码压缩包 方法二 使用git 1)git加入鼠标右键 2)git clone源码 2、VSCode出场 1)创建pyth…...
2024/4/30 4:00:30 - C# 构建可定时关闭的异步提示弹窗
C# 构建可定时关闭的异步提示弹窗 引言1、调用接口的实现2、自动定时窗口的实现 引言 我们在最常用最简单的提示弹框莫过于MessageBox.Show( )的方法了,但是使用久了之后,你会发现这个MessageBox并不是万能的,有事后并不想客户去点击&#x…...
2024/5/2 6:14:07 - 图像处理相关知识 —— 椒盐噪声
椒盐噪声是一种常见的图像噪声类型,它会在图像中随机地添加黑色(椒)和白色(盐)的像素点,使图像的质量降低。这种噪声模拟了在图像传感器中可能遇到的问题,例如损坏的像素或传输过程中的干扰。 椒…...
2024/5/5 8:37:08 - 【外汇早评】美通胀数据走低,美元调整
原标题:【外汇早评】美通胀数据走低,美元调整昨日美国方面公布了新一期的核心PCE物价指数数据,同比增长1.6%,低于前值和预期值的1.7%,距离美联储的通胀目标2%继续走低,通胀压力较低,且此前美国一季度GDP初值中的消费部分下滑明显,因此市场对美联储后续更可能降息的政策…...
2024/5/4 23:54:56 - 【原油贵金属周评】原油多头拥挤,价格调整
原标题:【原油贵金属周评】原油多头拥挤,价格调整本周国际劳动节,我们喜迎四天假期,但是整个金融市场确实流动性充沛,大事频发,各个商品波动剧烈。美国方面,在本周四凌晨公布5月份的利率决议和新闻发布会,维持联邦基金利率在2.25%-2.50%不变,符合市场预期。同时美联储…...
2024/5/4 23:54:56 - 【外汇周评】靓丽非农不及疲软通胀影响
原标题:【外汇周评】靓丽非农不及疲软通胀影响在刚结束的周五,美国方面公布了新一期的非农就业数据,大幅好于前值和预期,新增就业重新回到20万以上。具体数据: 美国4月非农就业人口变动 26.3万人,预期 19万人,前值 19.6万人。 美国4月失业率 3.6%,预期 3.8%,前值 3…...
2024/5/4 23:54:56 - 【原油贵金属早评】库存继续增加,油价收跌
原标题:【原油贵金属早评】库存继续增加,油价收跌周三清晨公布美国当周API原油库存数据,上周原油库存增加281万桶至4.692亿桶,增幅超过预期的74.4万桶。且有消息人士称,沙特阿美据悉将于6月向亚洲炼油厂额外出售更多原油,印度炼油商预计将每日获得至多20万桶的额外原油供…...
2024/5/4 23:55:17 - 【外汇早评】日本央行会议纪要不改日元强势
原标题:【外汇早评】日本央行会议纪要不改日元强势近两日日元大幅走强与近期市场风险情绪上升,避险资金回流日元有关,也与前一段时间的美日贸易谈判给日本缓冲期,日本方面对汇率问题也避免继续贬值有关。虽然今日早间日本央行公布的利率会议纪要仍然是支持宽松政策,但这符…...
2024/5/4 23:54:56 - 【原油贵金属早评】欧佩克稳定市场,填补伊朗问题的影响
原标题:【原油贵金属早评】欧佩克稳定市场,填补伊朗问题的影响近日伊朗局势升温,导致市场担忧影响原油供给,油价试图反弹。此时OPEC表态稳定市场。据消息人士透露,沙特6月石油出口料将低于700万桶/日,沙特已经收到石油消费国提出的6月份扩大出口的“适度要求”,沙特将满…...
2024/5/4 23:55:05 - 【外汇早评】美欲与伊朗重谈协议
原标题:【外汇早评】美欲与伊朗重谈协议美国对伊朗的制裁遭到伊朗的抗议,昨日伊朗方面提出将部分退出伊核协议。而此行为又遭到欧洲方面对伊朗的谴责和警告,伊朗外长昨日回应称,欧洲国家履行它们的义务,伊核协议就能保证存续。据传闻伊朗的导弹已经对准了以色列和美国的航…...
2024/5/4 23:54:56 - 【原油贵金属早评】波动率飙升,市场情绪动荡
原标题:【原油贵金属早评】波动率飙升,市场情绪动荡因中美贸易谈判不安情绪影响,金融市场各资产品种出现明显的波动。随着美国与中方开启第十一轮谈判之际,美国按照既定计划向中国2000亿商品征收25%的关税,市场情绪有所平复,已经开始接受这一事实。虽然波动率-恐慌指数VI…...
2024/5/4 23:55:16 - 【原油贵金属周评】伊朗局势升温,黄金多头跃跃欲试
原标题:【原油贵金属周评】伊朗局势升温,黄金多头跃跃欲试美国和伊朗的局势继续升温,市场风险情绪上升,避险黄金有向上突破阻力的迹象。原油方面稍显平稳,近期美国和OPEC加大供给及市场需求回落的影响,伊朗局势并未推升油价走强。近期中美贸易谈判摩擦再度升级,美国对中…...
2024/5/4 23:54:56 - 【原油贵金属早评】市场情绪继续恶化,黄金上破
原标题:【原油贵金属早评】市场情绪继续恶化,黄金上破周初中国针对于美国加征关税的进行的反制措施引发市场情绪的大幅波动,人民币汇率出现大幅的贬值动能,金融市场受到非常明显的冲击。尤其是波动率起来之后,对于股市的表现尤其不安。隔夜美国股市出现明显的下行走势,这…...
2024/5/4 18:20:48 - 【外汇早评】美伊僵持,风险情绪继续升温
原标题:【外汇早评】美伊僵持,风险情绪继续升温昨日沙特两艘油轮再次发生爆炸事件,导致波斯湾局势进一步恶化,市场担忧美伊可能会出现摩擦生火,避险品种获得支撑,黄金和日元大幅走强。美指受中美贸易问题影响而在低位震荡。继5月12日,四艘商船在阿联酋领海附近的阿曼湾、…...
2024/5/4 23:54:56 - 【原油贵金属早评】贸易冲突导致需求低迷,油价弱势
原标题:【原油贵金属早评】贸易冲突导致需求低迷,油价弱势近日虽然伊朗局势升温,中东地区几起油船被袭击事件影响,但油价并未走高,而是出于调整结构中。由于市场预期局势失控的可能性较低,而中美贸易问题导致的全球经济衰退风险更大,需求会持续低迷,因此油价调整压力较…...
2024/5/4 23:55:17 - 氧生福地 玩美北湖(上)——为时光守候两千年
原标题:氧生福地 玩美北湖(上)——为时光守候两千年一次说走就走的旅行,只有一张高铁票的距离~ 所以,湖南郴州,我来了~ 从广州南站出发,一个半小时就到达郴州西站了。在动车上,同时改票的南风兄和我居然被分到了一个车厢,所以一路非常愉快地聊了过来。 挺好,最起…...
2024/5/4 23:55:06 - 氧生福地 玩美北湖(中)——永春梯田里的美与鲜
原标题:氧生福地 玩美北湖(中)——永春梯田里的美与鲜一觉醒来,因为大家太爱“美”照,在柳毅山庄去寻找龙女而错过了早餐时间。近十点,向导坏坏还是带着饥肠辘辘的我们去吃郴州最富有盛名的“鱼头粉”。说这是“十二分推荐”,到郴州必吃的美食之一。 哇塞!那个味美香甜…...
2024/5/4 23:54:56 - 氧生福地 玩美北湖(下)——奔跑吧骚年!
原标题:氧生福地 玩美北湖(下)——奔跑吧骚年!让我们红尘做伴 活得潇潇洒洒 策马奔腾共享人世繁华 对酒当歌唱出心中喜悦 轰轰烈烈把握青春年华 让我们红尘做伴 活得潇潇洒洒 策马奔腾共享人世繁华 对酒当歌唱出心中喜悦 轰轰烈烈把握青春年华 啊……啊……啊 两…...
2024/5/4 23:55:06 - 扒开伪装医用面膜,翻六倍价格宰客,小姐姐注意了!
原标题:扒开伪装医用面膜,翻六倍价格宰客,小姐姐注意了!扒开伪装医用面膜,翻六倍价格宰客!当行业里的某一品项火爆了,就会有很多商家蹭热度,装逼忽悠,最近火爆朋友圈的医用面膜,被沾上了污点,到底怎么回事呢? “比普通面膜安全、效果好!痘痘、痘印、敏感肌都能用…...
2024/5/5 8:13:33 - 「发现」铁皮石斛仙草之神奇功效用于医用面膜
原标题:「发现」铁皮石斛仙草之神奇功效用于医用面膜丽彦妆铁皮石斛医用面膜|石斛多糖无菌修护补水贴19大优势: 1、铁皮石斛:自唐宋以来,一直被列为皇室贡品,铁皮石斛生于海拔1600米的悬崖峭壁之上,繁殖力差,产量极低,所以古代仅供皇室、贵族享用 2、铁皮石斛自古民间…...
2024/5/4 23:55:16 - 丽彦妆\医用面膜\冷敷贴轻奢医学护肤引导者
原标题:丽彦妆\医用面膜\冷敷贴轻奢医学护肤引导者【公司简介】 广州华彬企业隶属香港华彬集团有限公司,专注美业21年,其旗下品牌: 「圣茵美」私密荷尔蒙抗衰,产后修复 「圣仪轩」私密荷尔蒙抗衰,产后修复 「花茵莳」私密荷尔蒙抗衰,产后修复 「丽彦妆」专注医学护…...
2024/5/4 23:54:58 - 广州械字号面膜生产厂家OEM/ODM4项须知!
原标题:广州械字号面膜生产厂家OEM/ODM4项须知!广州械字号面膜生产厂家OEM/ODM流程及注意事项解读: 械字号医用面膜,其实在我国并没有严格的定义,通常我们说的医美面膜指的应该是一种「医用敷料」,也就是说,医用面膜其实算作「医疗器械」的一种,又称「医用冷敷贴」。 …...
2024/5/4 23:55:01 - 械字号医用眼膜缓解用眼过度到底有无作用?
原标题:械字号医用眼膜缓解用眼过度到底有无作用?医用眼膜/械字号眼膜/医用冷敷眼贴 凝胶层为亲水高分子材料,含70%以上的水分。体表皮肤温度传导到本产品的凝胶层,热量被凝胶内水分子吸收,通过水分的蒸发带走大量的热量,可迅速地降低体表皮肤局部温度,减轻局部皮肤的灼…...
2024/5/4 23:54:56 - 配置失败还原请勿关闭计算机,电脑开机屏幕上面显示,配置失败还原更改 请勿关闭计算机 开不了机 这个问题怎么办...
解析如下:1、长按电脑电源键直至关机,然后再按一次电源健重启电脑,按F8健进入安全模式2、安全模式下进入Windows系统桌面后,按住“winR”打开运行窗口,输入“services.msc”打开服务设置3、在服务界面,选中…...
2022/11/19 21:17:18 - 错误使用 reshape要执行 RESHAPE,请勿更改元素数目。
%读入6幅图像(每一幅图像的大小是564*564) f1 imread(WashingtonDC_Band1_564.tif); subplot(3,2,1),imshow(f1); f2 imread(WashingtonDC_Band2_564.tif); subplot(3,2,2),imshow(f2); f3 imread(WashingtonDC_Band3_564.tif); subplot(3,2,3),imsho…...
2022/11/19 21:17:16 - 配置 已完成 请勿关闭计算机,win7系统关机提示“配置Windows Update已完成30%请勿关闭计算机...
win7系统关机提示“配置Windows Update已完成30%请勿关闭计算机”问题的解决方法在win7系统关机时如果有升级系统的或者其他需要会直接进入一个 等待界面,在等待界面中我们需要等待操作结束才能关机,虽然这比较麻烦,但是对系统进行配置和升级…...
2022/11/19 21:17:15 - 台式电脑显示配置100%请勿关闭计算机,“准备配置windows 请勿关闭计算机”的解决方法...
有不少用户在重装Win7系统或更新系统后会遇到“准备配置windows,请勿关闭计算机”的提示,要过很久才能进入系统,有的用户甚至几个小时也无法进入,下面就教大家这个问题的解决方法。第一种方法:我们首先在左下角的“开始…...
2022/11/19 21:17:14 - win7 正在配置 请勿关闭计算机,怎么办Win7开机显示正在配置Windows Update请勿关机...
置信有很多用户都跟小编一样遇到过这样的问题,电脑时发现开机屏幕显现“正在配置Windows Update,请勿关机”(如下图所示),而且还需求等大约5分钟才干进入系统。这是怎样回事呢?一切都是正常操作的,为什么开时机呈现“正…...
2022/11/19 21:17:13 - 准备配置windows 请勿关闭计算机 蓝屏,Win7开机总是出现提示“配置Windows请勿关机”...
Win7系统开机启动时总是出现“配置Windows请勿关机”的提示,没过几秒后电脑自动重启,每次开机都这样无法进入系统,此时碰到这种现象的用户就可以使用以下5种方法解决问题。方法一:开机按下F8,在出现的Windows高级启动选…...
2022/11/19 21:17:12 - 准备windows请勿关闭计算机要多久,windows10系统提示正在准备windows请勿关闭计算机怎么办...
有不少windows10系统用户反映说碰到这样一个情况,就是电脑提示正在准备windows请勿关闭计算机,碰到这样的问题该怎么解决呢,现在小编就给大家分享一下windows10系统提示正在准备windows请勿关闭计算机的具体第一种方法:1、2、依次…...
2022/11/19 21:17:11 - 配置 已完成 请勿关闭计算机,win7系统关机提示“配置Windows Update已完成30%请勿关闭计算机”的解决方法...
今天和大家分享一下win7系统重装了Win7旗舰版系统后,每次关机的时候桌面上都会显示一个“配置Windows Update的界面,提示请勿关闭计算机”,每次停留好几分钟才能正常关机,导致什么情况引起的呢?出现配置Windows Update…...
2022/11/19 21:17:10 - 电脑桌面一直是清理请关闭计算机,windows7一直卡在清理 请勿关闭计算机-win7清理请勿关机,win7配置更新35%不动...
只能是等着,别无他法。说是卡着如果你看硬盘灯应该在读写。如果从 Win 10 无法正常回滚,只能是考虑备份数据后重装系统了。解决来方案一:管理员运行cmd:net stop WuAuServcd %windir%ren SoftwareDistribution SDoldnet start WuA…...
2022/11/19 21:17:09 - 计算机配置更新不起,电脑提示“配置Windows Update请勿关闭计算机”怎么办?
原标题:电脑提示“配置Windows Update请勿关闭计算机”怎么办?win7系统中在开机与关闭的时候总是显示“配置windows update请勿关闭计算机”相信有不少朋友都曾遇到过一次两次还能忍但经常遇到就叫人感到心烦了遇到这种问题怎么办呢?一般的方…...
2022/11/19 21:17:08 - 计算机正在配置无法关机,关机提示 windows7 正在配置windows 请勿关闭计算机 ,然后等了一晚上也没有关掉。现在电脑无法正常关机...
关机提示 windows7 正在配置windows 请勿关闭计算机 ,然后等了一晚上也没有关掉。现在电脑无法正常关机以下文字资料是由(历史新知网www.lishixinzhi.com)小编为大家搜集整理后发布的内容,让我们赶快一起来看一下吧!关机提示 windows7 正在配…...
2022/11/19 21:17:05 - 钉钉提示请勿通过开发者调试模式_钉钉请勿通过开发者调试模式是真的吗好不好用...
钉钉请勿通过开发者调试模式是真的吗好不好用 更新时间:2020-04-20 22:24:19 浏览次数:729次 区域: 南阳 > 卧龙 列举网提醒您:为保障您的权益,请不要提前支付任何费用! 虚拟位置外设器!!轨迹模拟&虚拟位置外设神器 专业用于:钉钉,外勤365,红圈通,企业微信和…...
2022/11/19 21:17:05 - 配置失败还原请勿关闭计算机怎么办,win7系统出现“配置windows update失败 还原更改 请勿关闭计算机”,长时间没反应,无法进入系统的解决方案...
前几天班里有位学生电脑(windows 7系统)出问题了,具体表现是开机时一直停留在“配置windows update失败 还原更改 请勿关闭计算机”这个界面,长时间没反应,无法进入系统。这个问题原来帮其他同学也解决过,网上搜了不少资料&#x…...
2022/11/19 21:17:04 - 一个电脑无法关闭计算机你应该怎么办,电脑显示“清理请勿关闭计算机”怎么办?...
本文为你提供了3个有效解决电脑显示“清理请勿关闭计算机”问题的方法,并在最后教给你1种保护系统安全的好方法,一起来看看!电脑出现“清理请勿关闭计算机”在Windows 7(SP1)和Windows Server 2008 R2 SP1中,添加了1个新功能在“磁…...
2022/11/19 21:17:03 - 请勿关闭计算机还原更改要多久,电脑显示:配置windows更新失败,正在还原更改,请勿关闭计算机怎么办...
许多用户在长期不使用电脑的时候,开启电脑发现电脑显示:配置windows更新失败,正在还原更改,请勿关闭计算机。。.这要怎么办呢?下面小编就带着大家一起看看吧!如果能够正常进入系统,建议您暂时移…...
2022/11/19 21:17:02 - 还原更改请勿关闭计算机 要多久,配置windows update失败 还原更改 请勿关闭计算机,电脑开机后一直显示以...
配置windows update失败 还原更改 请勿关闭计算机,电脑开机后一直显示以以下文字资料是由(历史新知网www.lishixinzhi.com)小编为大家搜集整理后发布的内容,让我们赶快一起来看一下吧!配置windows update失败 还原更改 请勿关闭计算机&#x…...
2022/11/19 21:17:01 - 电脑配置中请勿关闭计算机怎么办,准备配置windows请勿关闭计算机一直显示怎么办【图解】...
不知道大家有没有遇到过这样的一个问题,就是我们的win7系统在关机的时候,总是喜欢显示“准备配置windows,请勿关机”这样的一个页面,没有什么大碍,但是如果一直等着的话就要两个小时甚至更久都关不了机,非常…...
2022/11/19 21:17:00 - 正在准备配置请勿关闭计算机,正在准备配置windows请勿关闭计算机时间长了解决教程...
当电脑出现正在准备配置windows请勿关闭计算机时,一般是您正对windows进行升级,但是这个要是长时间没有反应,我们不能再傻等下去了。可能是电脑出了别的问题了,来看看教程的说法。正在准备配置windows请勿关闭计算机时间长了方法一…...
2022/11/19 21:16:59 - 配置失败还原请勿关闭计算机,配置Windows Update失败,还原更改请勿关闭计算机...
我们使用电脑的过程中有时会遇到这种情况,当我们打开电脑之后,发现一直停留在一个界面:“配置Windows Update失败,还原更改请勿关闭计算机”,等了许久还是无法进入系统。如果我们遇到此类问题应该如何解决呢࿰…...
2022/11/19 21:16:58 - 如何在iPhone上关闭“请勿打扰”
Apple’s “Do Not Disturb While Driving” is a potentially lifesaving iPhone feature, but it doesn’t always turn on automatically at the appropriate time. For example, you might be a passenger in a moving car, but your iPhone may think you’re the one dri…...
2022/11/19 21:16:57